@BoRRoZ
Thanks for your reply. Here you go!
- show output of your TTN application console when device is joining (trying too)
The output of the sketch in Serial Monitor
Click to see the full output
-- STATUS
EUI: 0004A30B001F9A2B
Battery: 3325
AppEUI: 70B3D57ED00168DA
DevEUI: 0004A30B001F9A2B
Data Rate: 0
RX Delay 1: 1000
RX Delay 2: 2000
-- JOIN
Model: RN2483
Version: 1.0.4
Sending: mac set deveui 0004A30B001F9A2B
Sending: mac set adr off
Sending: mac set deveui 0004A30B001F9A2B
Sending: mac set appeui 70B3D57ED00168DA
Sending: mac set appkey D861DB7C58D63473D48A6887FAD93A68
Sending: mac save
Sending: mac set rx2 3 869525000
Sending: mac set ch drrange 1 0 6
Sending: mac set ch dcycle 0 799
Sending: mac set ch dcycle 1 799
Sending: mac set ch dcycle 2 799
Sending: mac set ch dcycle 3 799
Sending: mac set ch freq 3 867100000
Sending: mac set ch drrange 3 0 5
Sending: mac set ch status 3 on
Sending: mac set ch dcycle 4 799
Sending: mac set ch freq 4 867300000
Sending: mac set ch drrange 4 0 5
Sending: mac set ch status 4 on
Sending: mac set ch dcycle 5 799
Sending: mac set ch freq 5 867500000
Sending: mac set ch drrange 5 0 5
Sending: mac set ch status 5 on
Sending: mac set ch dcycle 6 799
Sending: mac set ch freq 6 867700000
Sending: mac set ch drrange 6 0 5
Sending: mac set ch status 6 on
Sending: mac set ch dcycle 7 799
Sending: mac set ch freq 7 867900000
Sending: mac set ch drrange 7 0 5
Sending: mac set ch status 7 on
Sending: mac set pwridx 1
Sending: mac set retx 7
Sending: mac set dr 5
Sending: mac join otaa
Join not accepted: denied
Check your coverage, keys and backend status.
Sending: mac join otaa
Join not accepted: denied
Check your coverage, keys and backend status.
Sending: mac join otaa
Join not accepted: denied
Check your coverage, keys and backend status.
Sending: mac join otaa
Response is not OK: no_free_ch
Send join command failed
Sending: mac join otaa
Response is not OK: no_free_ch
Send join command failed
Sending: mac join otaa
Response is not OK: no_free_ch
The output of TTN Console
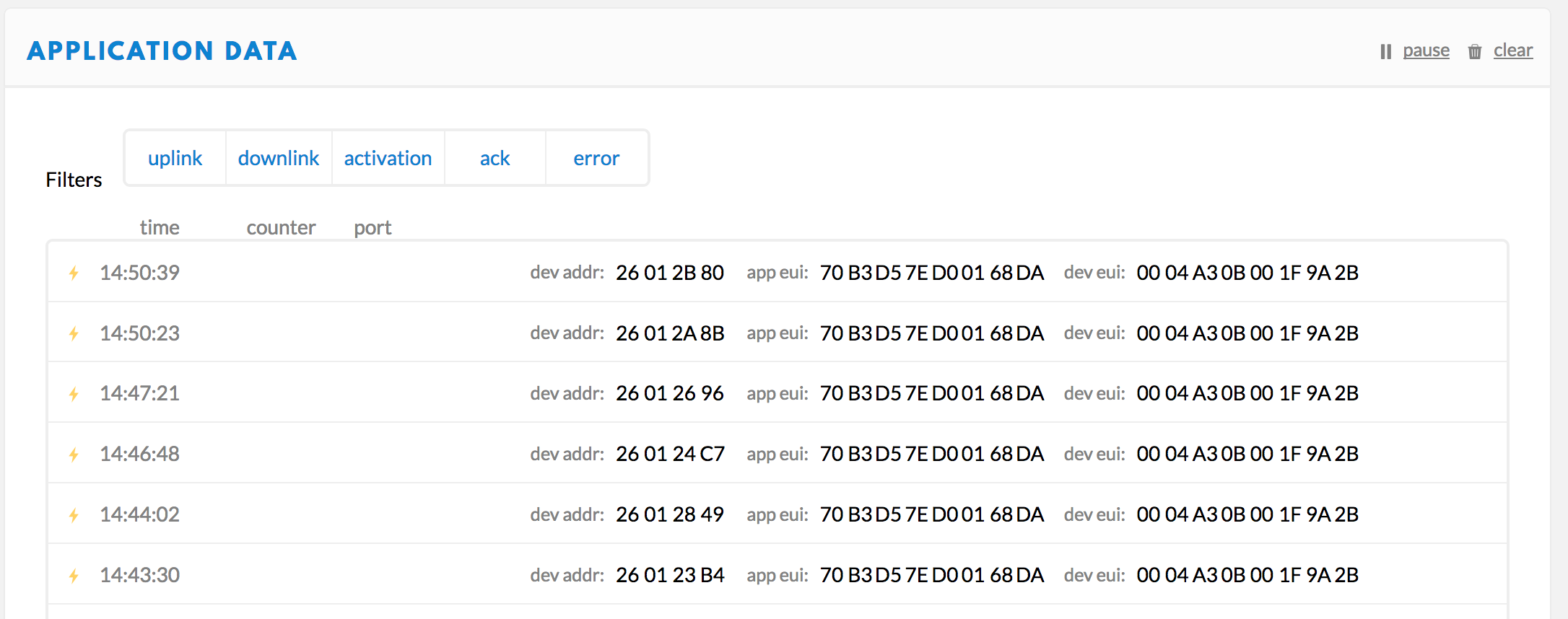
- show your full FORMATTED sketch or a link to it
Simple LoRa sketch
Click to see the full code
/*
* Compatible with:
* SODAQ MBILI
* SODAQ Autonomo
* SODAQ ONE
* SODAQ ONE BETA
* SODAQ EXPLORER
*/
#include "Arduino.h"
#include <Sodaq_RN2483.h>
#define debugSerial SERIAL_PORT_MONITOR
#if defined(ARDUINO_AVR_SODAQ_MBILI)
#define loraSerial Serial1
#define BEE_VCC 20
#elif defined(ARDUINO_SODAQ_AUTONOMO) || defined(ARDUINO_SODAQ_ONE) || defined(ARDUINO_SODAQ_ONE_BETA)
#define loraSerial Serial1
#elif defined(ARDUINO_SODAQ_EXPLORER)
#define loraSerial Serial2
#else
// please select a sodaq board
debugSerial.println("Please select a sodaq board!!");
#endif
// ABP
const uint8_t devAddr[4] = { 0x00, 0x00, 0x00, 0x00 };
const uint8_t appSKey[16] = { 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00 };
const uint8_t nwkSKey[16] = { 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00, 0x00 };
// OTAA
uint8_t DevEUI[8] = { 0x00, 0x04, 0xA3, 0x0B, 0x00, 0x1F, 0x9A, 0x2B };
uint8_t AppEUI[8] = { 0x70, 0xB3, 0xD5, 0x7E, 0xD0, 0x01, 0x68, 0xDA };
uint8_t AppKey[16] = { 0xD8, 0x61, 0xDB, 0x7C, 0x58, 0xD6, 0x34, 0x73, 0xD4, 0x8A, 0x68, 0x87, 0xFA, 0xD9, 0x3A, 0x68 };
void setupLoRaABP(){
if (LoRaBee.initABP(loraSerial, devAddr, appSKey, nwkSKey, false))
{
debugSerial.println("Communication to LoRaBEE successful.");
}
else
{
debugSerial.println("Communication to LoRaBEE failed!");
}
}
void setupLoRaOTAA(){
if (LoRaBee.initOTA(loraSerial, DevEUI, AppEUI, AppKey, false))
{
debugSerial.println("Communication to LoRaBEE successful.");
}
else
{
debugSerial.println("OTAA Setup failed!");
}
}
void setup() {
//Power up the LoRaBEE
#if defined(ARDUINO_AVR_SODAQ_MBILI) || defined(ARDUINO_SODAQ_AUTONOMO)
pinMode(BEE_VCC, OUTPUT);
digitalWrite(BEE_VCC, HIGH);
#endif
delay(3000);
while ((!SerialUSB) && (millis() < 10000)){
// Wait 10 seconds for the Serial Monitor
}
//Set baud rate
debugSerial.begin(57600);
loraSerial.begin(LoRaBee.getDefaultBaudRate());
// Debug output from LoRaBee
// LoRaBee.setDiag(debugSerial); // optional
//connect to the LoRa Network
setupLoRa();
}
void setupLoRa(){
// ABP
// setupLoRaABP();
// OTAA
setupLoRaOTAA();
LoRaBee.setSpreadingFactor(9);
}
void sendPacket(String packet){
switch (LoRaBee.send(1, (uint8_t*)packet.c_str(), packet.length()))
{
case NoError:
debugSerial.println("Successful transmission.");
break;
case NoResponse:
debugSerial.println("There was no response from the device.");
setupLoRa();
break;
case Timeout:
debugSerial.println("Connection timed-out. Check your serial connection to the device! Sleeping for 20sec.");
delay(20000);
break;
case PayloadSizeError:
debugSerial.println("The size of the payload is greater than allowed. Transmission failed!");
break;
case InternalError:
debugSerial.println("Oh No! This shouldn't happen. Something is really wrong! Try restarting the device!\r\nThe network connection will reset.");
setupLoRa();
break;
case Busy:
debugSerial.println("The device is busy. Sleeping for 10 extra seconds.");
delay(10000);
break;
case NetworkFatalError:
debugSerial.println("There is a non-recoverable error with the network connection. You should re-connect.\r\nThe network connection will reset.");
setupLoRa();
break;
case NotConnected:
debugSerial.println("The device is not connected to the network. Please connect to the network before attempting to send data.\r\nThe network connection will reset.");
setupLoRa();
break;
case NoAcknowledgment:
debugSerial.println("There was no acknowledgment sent back!");
// When you this message you are probaly out of range of the network.
break;
default:
break;
}
}
void loop() {
// put your main code here, to run repeatedly:
String packet = "SODAQ";
sendPacket(packet);
delay(10000);
}
SendOTAA sketch
Click to see the full code
#include <TheThingsNetwork.h>
// Set your AppEUI and AppKey
const char *appEui = "70B3D57ED00168DA";
const char *appKey = "D861DB7C58D63473D48A6887FAD93A68";
#define loraSerial Serial2
#define debugSerial SerialUSB
// Replace REPLACE_ME with TTN_FP_EU868 or TTN_FP_US915
#define freqPlan TTN_FP_EU868
TheThingsNetwork ttn(loraSerial, debugSerial, freqPlan);
void setup()
{
loraSerial.begin(57600);
debugSerial.begin(9600);
// Wait a maximum of 10s for Serial Monitor
while (!debugSerial && millis() < 10000)
;
debugSerial.println("-- STATUS");
ttn.showStatus();
debugSerial.println("-- JOIN");
ttn.join(appEui, appKey);
ttn.onMessage(message);
pinMode(LED_BUILTIN, OUTPUT);
}
void loop()
{
uint16_t temp = getTemperature();
byte payload[2];
payload[0] = highByte(temp);
payload[1] = lowByte(temp);
ttn.sendBytes(payload, sizeof(payload));
// Delay between readings - 10 000 = 10 secons
delay(10000);
}
uint16_t getTemperature()
{
//10mV per C, 0C is 500mV
float mVolts = (float)analogRead(TEMP_SENSOR) * 3300.0 / 1023.0;
int temp = (mVolts - 500) * 10;
debugSerial.print((mVolts - 500) / 10);
debugSerial.println(" Celcius");
return int(temp);
}
void message(const uint8_t *payload, size_t size, port_t port) {
if (payload[0] == 0) {
digitalWrite(LED_BUILTIN, LOW);
} else if (payload[0] == 1) {
digitalWrite(LED_BUILTIN, HIGH);
}
}
-
what type of gateway do you use (you don’t ‘connect’ to a gateway- it’s not wifi)
I don’t know the type of gateway, it’s a public one.
Yeah, yeah, I know you don’t connect. Messages are passed by the gateway to TTN, where they are refused a join.
-
what are the used antenna’s and what is the distance between GW and node
Which antenna’s are you referring to? The Sodaq Explorer board has an embedded (PCB) LoRa antenna, that’s all I can find on the Sodaq website.The gateway’s antenna is unknown to me.
Distance between gateway and node is 500 meter
P.S.: I leave the keys in the code/output for clarity. Will change them shortly.