Sure, but i don’t use LMIC nor TTN network, since all i need to monitor are my rooms temp/humidity, i have my own custom gateway pushing from lora >> udp >> influxdb (grafana) self hosted.
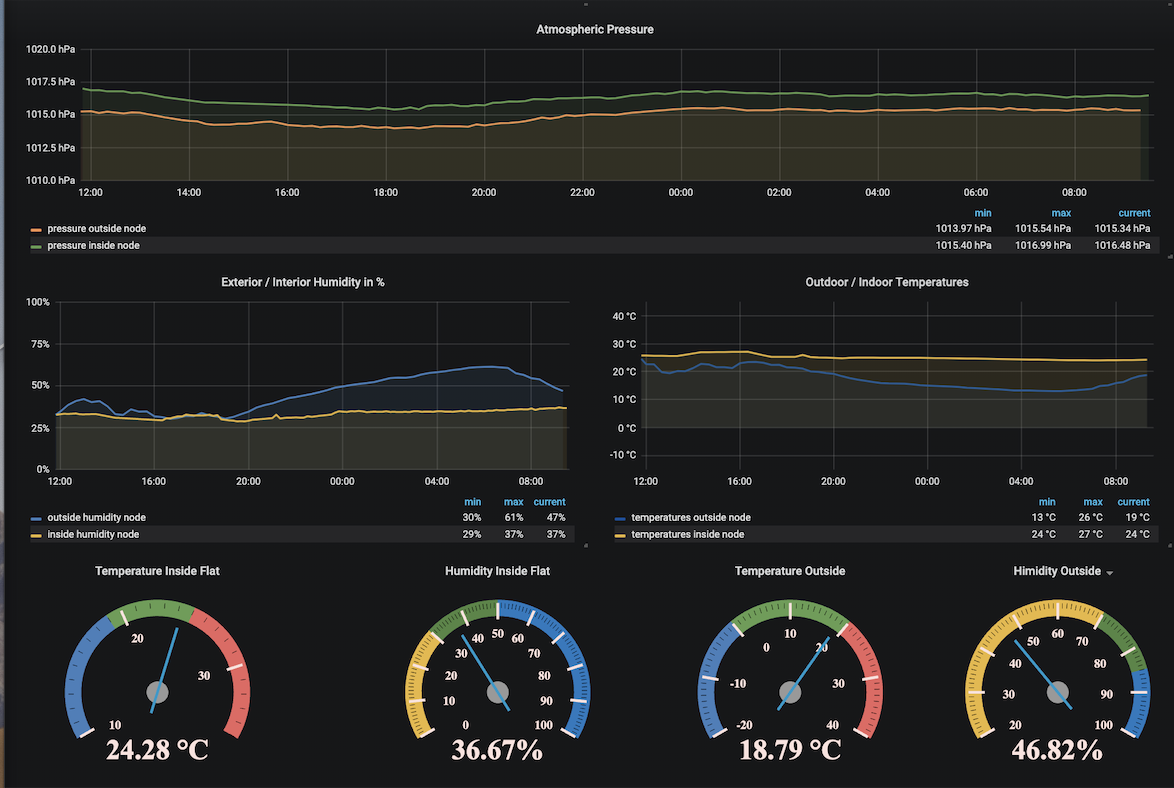
So you will need to adapt the code and change LoRa lib to LMIC if you wanna join TTN network 
So there you go:
#include <SPI.h>
#include <LoRa.h>
#include <Wire.h>
#include <Arduino.h>
#include <rBase64.h>
#include <ArduinoJson.h>
#include <Adafruit_Sensor.h>
#include <Adafruit_BME280.h>
#include "PinChangeInterrupt.h"
// BME280
#define SEALEVELPRESSURE_HPA (1013.25)
//LORA
#define NSS 10
#define RST A0
#define DI0 2
#define SCK 13
#define MISO 12
#define MOSI 11
#define BAND 868E6
#define BLUE_LED 13
#define SF_FACTOR 7
const int drvPin = A2;
const int DONEPin = A1;
bool sendData = true;
Adafruit_BME280 bme;
void do_smth(void) {
sendData = true;
}
void setup() {
if (! bme.begin(0x76)) {
while (1);
}
bme.setSampling(Adafruit_BME280::MODE_FORCED,
Adafruit_BME280::SAMPLING_X1, // temperature
Adafruit_BME280::SAMPLING_X1, // pressure
Adafruit_BME280::SAMPLING_X1, // humidity
Adafruit_BME280::FILTER_OFF );
delay(10);
LoRa.setPins(SS,RST,DI0);
if (!LoRa.begin(BAND)) {
while (1);
}
LoRa.setSpreadingFactor(SF_FACTOR);
// set pin to input with a pullup, DONE to output
pinMode(drvPin, INPUT_PULLUP);
pinMode(DONEPin, OUTPUT);
attachPCINT(digitalPinToPCINT(drvPin), do_smth, FALLING); // DRV goes LOW, when TPL time has elapsed
//ENABLE SLEEP - this enables the sleep mode
SMCR |= (1 << 2); //power down mode
SMCR |= 1;//enable sleep
}
void goToSleep()
{
LoRa.sleep();
ADCSRA &= ~(1 << 7);
//BOD DISABLE - this must be called right before the __asm__ sleep instruction
MCUCR |= (3 << 5); //set both BODS and BODSE at the same time
MCUCR = (MCUCR & ~(1 << 5)) | (1 << 6); //then set the BODS bit and clear the BODSE bit at the same time
__asm__ __volatile__("sleep");//in line assembler to go to sleep
}
void loop() {
if (sendData)
{
bme.takeForcedMeasurement();
const size_t capacity = JSON_OBJECT_SIZE(4);
DynamicJsonDocument doc(capacity);
String output;
doc["t"] = bme.readTemperature();
doc["h"] = bme.readHumidity();
doc["p"] = bme.readPressure() / 100.0F;
serializeJson(doc, output);
// For local test puropse, it's enough, to make it prod ready, use AES !!!
rbase64.encode(output);
LoRa.beginPacket();
LoRa.print(rbase64.result());
LoRa.endPacket();
sendData = false; //so do_smth() can alter state;
digitalWrite(DONEPin, HIGH);
delay(1);
digitalWrite(DONEPin, LOW);
goToSleep();
}
}
For sure, you can make much prettier with some more love and patience.
Regarding battery consumption, i put a 2.5 ohm resistor in serie and tried to measure the voltage drop, thus deducing the current.
With BME280 and rfm95W WITHOUT sleep mode ~2.3 mA
With BME280 and rfm95W WITH sleep mode , i can’t measure as my UT61E give me 0.00 mV, thus, all i know is that i’m under 4 uA
PS: I would like to thanks all those helped me, but a special thanks to @jenertsA for helping on the TPL code !